Turn your HTML5 games into desktop applications using Electron and distribute them – Part 1: Windows
Talking about Game development, HTML5 and Javascript.
Did you develop a HTML5 game and now you want to distribute it as a desktop application for Windows, macOS or Linux? Thanks to Electron, now you can.
Electron is a framework for creating native applications with web technologies like JavaScript, HTML, and CSS. Since HTML5 games are just web pages powered by JavaScript, HTML, and CSS, we can turn them into native applications.
This guide describes the simplest and fastest way to build a distributable Windows app starting from 4096 HTML5 game I built with Phaser.
This is also the game you will learn to build from scratch following my book HTML5 Cross Platform Game Development Using Phaser 3. The book has a lot of positive feedbacks and is going to receive another free update so it’s a must have if you want to learn HTML5 game development.
As said, this is the fastest way to turn your HTML5 game into a native desktop app, so we are going to install as few libraries as possible.
We have to install Node.js on our machine. You should already have installed it, especially if you are publishing your HTML5 games to mobile stores, anyway in your Command Prompt, PowerShell or any other CLI of you choice, write:
node -v
If you get an error, or have a version older than the one currently available on the official download page, then download and install it.
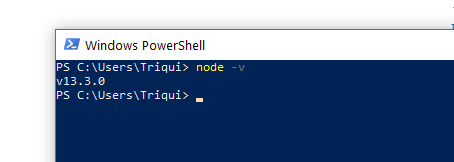
Once you have Node.js installed and working, create an empty folder and inside this folder create a file called package.json
with this content:
{
"name": "myElectronGame",
"version": "1.0.0",
"main": "index.js",
"scripts": {
"start": "electron ."
}
}
We are now ready to install Electron. We are going to install it as a development dependency, so we can have different HTML5 games turned into desktop apps each one with its Electron version.
From your CLI, go to the same folder containing the newly created package.json
, and enter:
npm install --save-dev electron
You should see something like this:
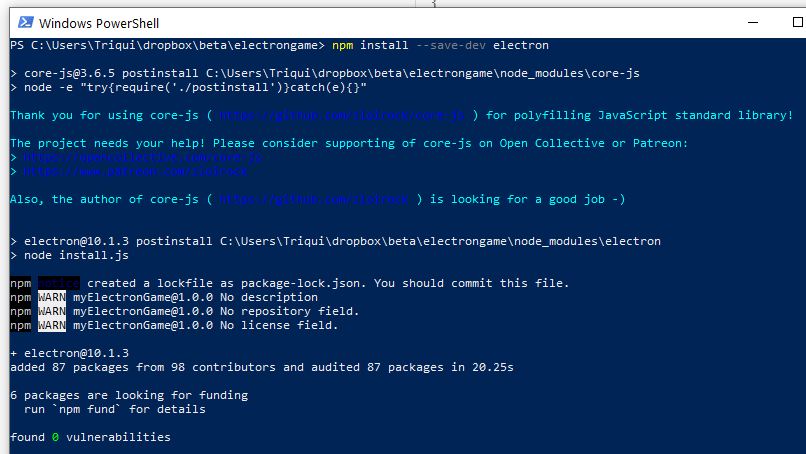
Now if you open package.json
you should see these new three lines:
{
"name": "myElectronGame",
"version": "1.0.0",
"main": "index.js",
"scripts": {
"start": "electron ."
},
"devDependencies": {
"electron": "^10.1.3"
}
}
Now we need, in the same folder, a JavaScript file called index.js
, as we defined at line 4, with this content:
const { app, BrowserWindow } = require('electron')
function createWindow() {
const win = new BrowserWindow({
width: 600,
height: 800,
webPreferences: {
nodeIntegration: true
}
})
win.loadFile('index.html');
}
app.whenReady().then(createWindow)
The customizable part is pretty straightforward, at lines 5 and 6 you will set your window width and height.
Now you have to copy your game in the same folder, which now will look this way:
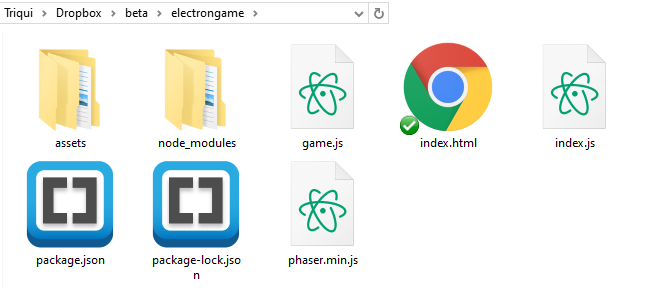
node_modules
folder has been created by Electron installation, as well as package-lock.json
.
package.json
and index.js
are the files we created some minutes ago, while the remaining files and folders are part of my 4096 game.
Ready to turn the HTML5 game into a desktop app? From the CLI, enter:
npm start
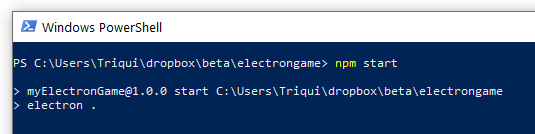
And you should see your game running in a window:
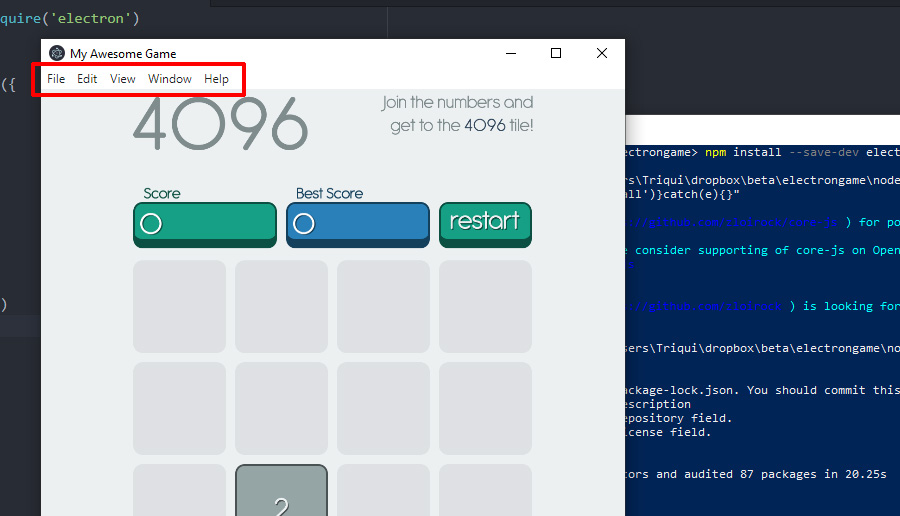
Obviously, we do not want those annoying menu bar, so we have to add one line to index.js
file:
const { app, BrowserWindow } = require('electron')
function createWindow() {
const win = new BrowserWindow({
width: 600,
height: 800,
webPreferences: {
nodeIntegration: true
}
})
win.setMenuBarVisibility(false);
win.loadFile('index.html');
}
app.whenReady().then(createWindow)
Once you added line 11, launch the game from the CLI again and you will see the window without any menu bar.
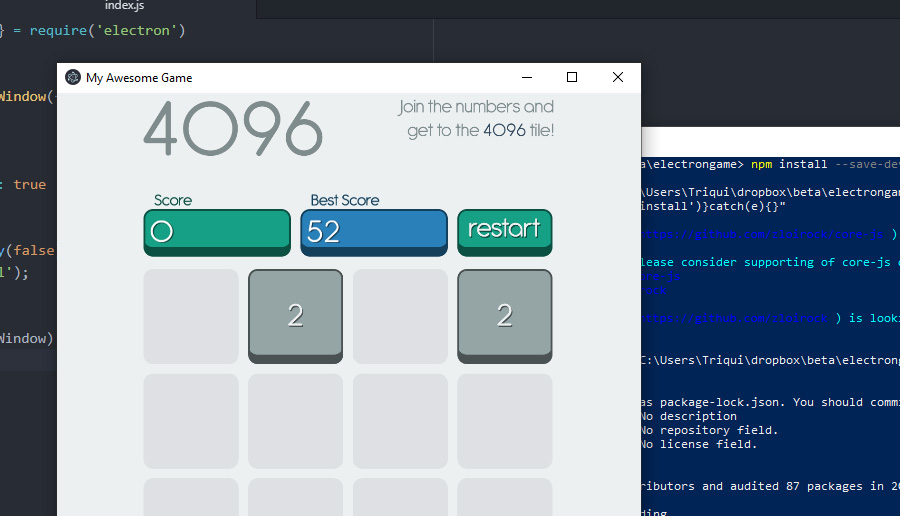
We are now able to run a HTML5 game as a desktop app, but we don’t know yet how to distribute it.
In order to distribute your game, you have to copy all game files, as well as index.js
and package.json
to this folder:
node_modules/electron/dist/resources/app
Which now will look this way:
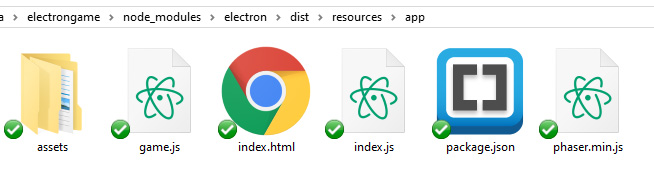
You are now able to distribute the dist
folder wich will look this way:
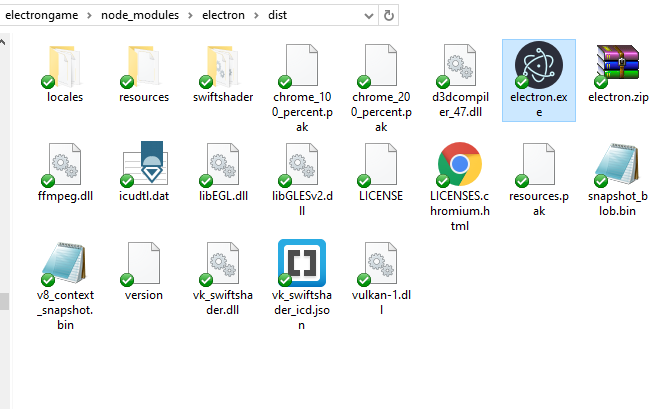
You can run your game by double clicking electron.exe
executable file. Copy dist
folder anywhere and you will be able to run the game as a desktop app.
There is something more to do: we want to create an installer, change the name and the icon of the game, and protect our game which is now exposed.
We’ll cover these features in next step, meanwhile download the entire project and try to build and run your HTML5 games as native desktop apps.
Never miss an update! Subscribe, and I will bother you by email only when a new game or full source code comes out.