Talking about Tipsy Tower game, C#, Game development and Unity3D.
If you enjoyed the Tipsy Tower prototype made with Construct here I am with the Unity version, powered with the free platformer art tileset provided by Game Art 2D Let’s start from the result, here’s what I built: Click to drop the crate. Now it’s time to have a look at my Unity scene: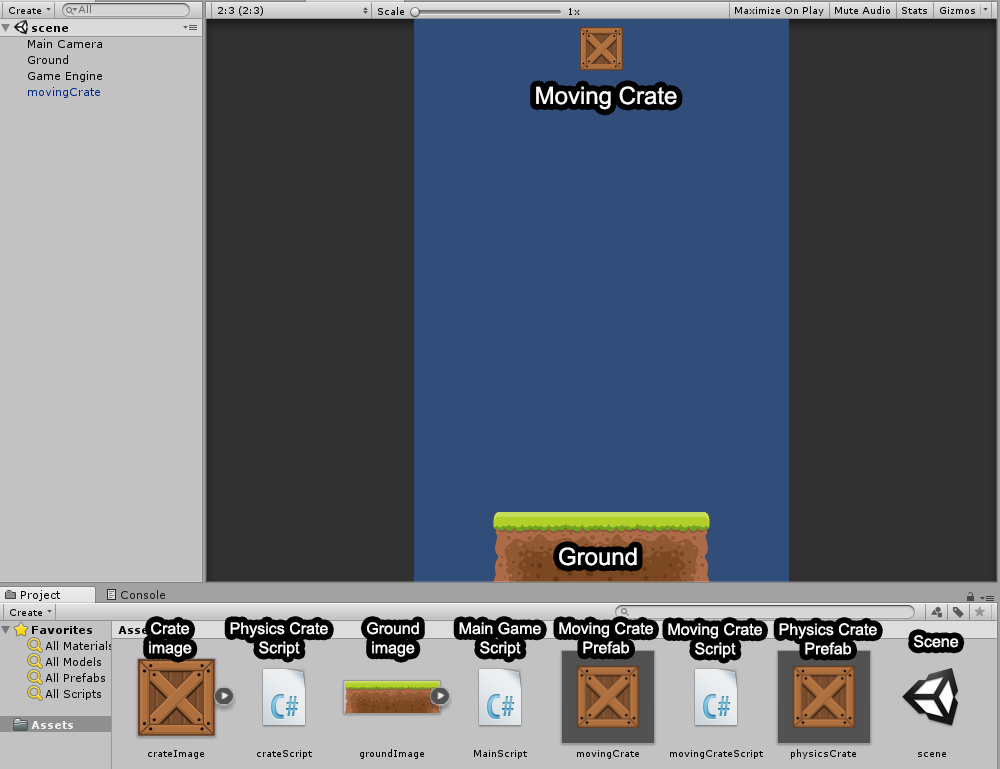
crateScript.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class crateScript : MonoBehaviour {
// this function is executed when the game object become ivisible, in this case
// when it leaves the stage
void OnBecameInvisible(){
// destroying game object
Destroy (gameObject);
}
}
Now, the moving crate script, which is only a non phyiscs driven crate moving from left to right:
movingCrateScript.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class movingCrateScript : MonoBehaviour {
// this function is executed at each frame
void Update () {
// since we can’t just change the x position of a game object, we have to create a position which is the same
// as the original game object position
Vector3 newPosition = transform.position;
// this is how we can set a tween, using PingPong on a variable (in this case the time),
// so that it is never larger than length and never smaller than 0.
// The returned value will move back and forth between 0 and 4.4f
newPosition.x = Mathf.PingPong (Time.time * 5.0f, 4.4f) – 2.2f;
// and finally we apply new position to game object’s position
transform.position = newPosition;
}
}
The main script waits for player input, handles physics crate creation and hides the moving crate.
MainScript.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MainScript : MonoBehaviour {
// crateObject is the physic crate prefab
public GameObject crateObject;
// a variable used only inside this class, for PRIVATE use.
// canDrop is true if the player can drop a crate, false when the player can’t
private bool canDrop = true;
// this function is executed at each frame
void Update () {
// checking for player input AND canDrop to be true.
// it means the player can drop a crate
if (Input.GetButtonDown (“Fire1”) && canDrop) {
// set canDrop to false
canDrop = false;
// find an object called “movinCrate” and store it into movingCrate variable
GameObject movingCrate = GameObject.Find (“movingCrate”);
// calling cangeAlpha function to set movingCrate’s alpha to zero (completely transparent)
changeAlpha (movingCrate, 0f);
// instantiate a crateObject instance and assign it to physicsCrate variable
GameObject physicsCrate = Instantiate(crateObject);
// set physicsCrate position as the same of movingCrate position
physicsCrate.transform.position = movingCrate.transform.position;
// starting a coroutine – set a s waitForCrate function.
// the interesting thing of a coroutine is it can be paused at any point using the yield statement
StartCoroutine (waitForCrate(movingCrate));
}
}
// the IEnumerator allows the program to yield things like the WaitForSeconds function,
// which lets you tell the script to wait without hogging the CPU
IEnumerator waitForCrate(GameObject crate){
// this means “wait 2 seconds”
yield return new WaitForSeconds(2);
// setting canDrop to true again, now the player can drop another crate
canDrop = true;
// setting moving crate alpha to 1
changeAlpha (crate, 1f);
}
// this function changes the alpha of a game object
void changeAlpha(GameObject sprite, float alpha){
// since we can’t just change the alpha, we have to create a color which is the same
// as the original game object color
Color color = sprite.GetComponent().material.color;
// then we change color’s alpha
color.a = alpha;
// and finally we apply new color to game object’s color
sprite.GetComponent().material.color = color;
}
}
And despite there was some code to write, it was easy and fun to make the prototype. Next time I will show you how to do it with Phaser, then we’ll improve the prototype to check for the highest crate, meanwhile download the Unity project. Never miss an update! Subscribe, and I will bother you by email only when a new game or full source code comes out.