Talking about HTML5, Javascript, Phaser and TypeScript.
As promised, here we are with step 3 of the migration from JavaScript to TypeScript in the building of HTML5 games using Phaser.
In step 1 we installed and set up Node.js.
In step 2 I covered npm and Parcel.
Now it’s time to start coding in TypeScript.
First we have to install TypeScript with npm, with
npm install typescript -g
And now you have TypeScript installed.
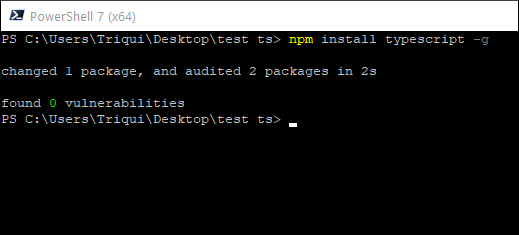
What now? Let’s create a TypeScript file called script.ts
with this content:
s = 'Hello World';
console.log(s);
Then let’s run it with
tsc script.ts
And you will get errors:
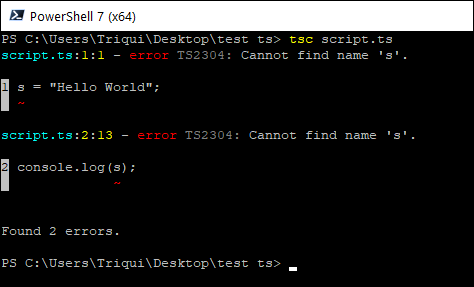
A valid JavaScript file, but it doesn’t work in TypeScript because variables must be declared, this way:
const s = 'Hello World';
console.log(s);
or even better, this way:
const s: string = 'Hello World';
console.log(s);
With type declaration.
Now run again
tsc script.ts
Then
npm script.js
And this time you’ll get a result:
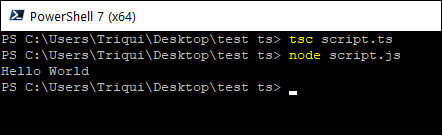
This is because TypeScript created a valid JavaScript file which has been executed by Node.js. Look at the generated JavaScript file:
var s = 'Hello World';
console.log(s);
So, from now on, we can say we will be coding in a “cool” JavaScript, which will be translated in a “ugly” JavaScript and executed.
Time to put all together, let’s create a project as seen in Phaser and the migration from JavaScript to TypeScript – Step 2 – npm and Parcel with
npm init -y
As you should know, a file called package.json
is created:
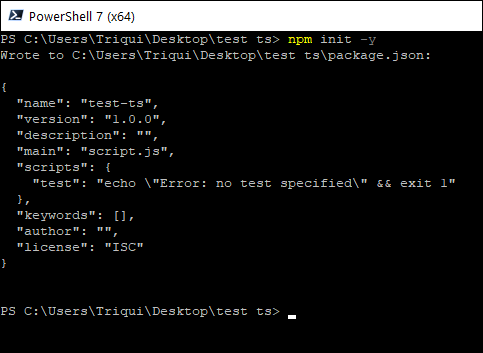
Let’s edit it, adding one line:
{
"name": "test-ts",
"version": "1.0.0",
"description": "",
"main": "script.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"parceltest": "parcel index.html"
},
"keywords": [],
"author": "",
"license": "ISC"
}
Now let’s create index.html
:
<html>
<head>
<title>Hello World</title>
</head>
<body>
<div id = "main"></div>
<script src = "script.ts"></script>
</body>
</html>
Then change script.ts
this way:
const s: string = "Hello World";
document.querySelector("#main").innerHTML = s;
Now if we go to command line and write
npm run test
We’ll run the “test” script inside package.json
:
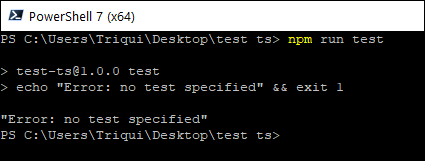
So if you call “parceltest” script…
npm run parceltest
It will run Parcel on index.html files, analyse and convert all TypeScript files and run the server as we saw in previous step:
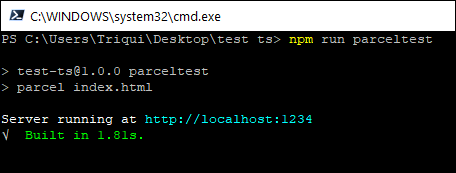
And if we go to http://localhost:1234/
, we’ll see the project running:
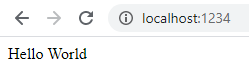
Our script.ts
has also been changed:
{
"name": "test-ts",
"version": "1.0.0",
"description": "",
"main": "script.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"parceltest": "parcel index.html"
},
"keywords": [],
"author": "",
"license": "ISC",
"devDependencies": {
"typescript": "^4.3.2"
}
}
Now let’s intall Phaser:
npm install phaser --save
And now Phaser is installed:
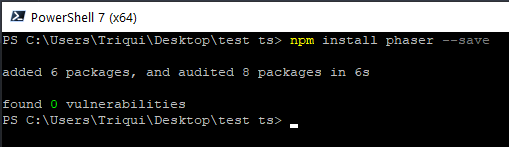
package.json changed again:
{
"name": "test-ts",
"version": "1.0.0",
"description": "",
"main": "script.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"parceltest": "parcel index.html"
},
"keywords": [],
"author": "",
"license": "ISC",
"devDependencies": {
"typescript": "^4.3.2"
},
"dependencies": {
"phaser": "^3.55.2"
}
}
Now change the content of index.html
just like in previous example, but remember to use ts
rather than js
:
<!DOCTYPE html>
<html>
<head>
<script src="game.ts"></script>
</head>
</html>
This is game.ts
:
import Phaser from 'phaser'
import { GameScene } from "./gameScene";
const config: Phaser.Types.Core.GameConfig = {
type: Phaser.AUTO,
width: 640,
height: 320,
scene: GameScene
};
new Phaser.Game(config);
And this is gameScene.ts
:
export class GameScene extends Phaser.Scene {
constructor() {
super({ key: "GameScene" });
}
create() {
this.add.text(10, 10, "HELLO WORLD");
}
}
Build the project again with
npm run parceltest
And here is our Phaser project up and running at http://localhost:1234/
:
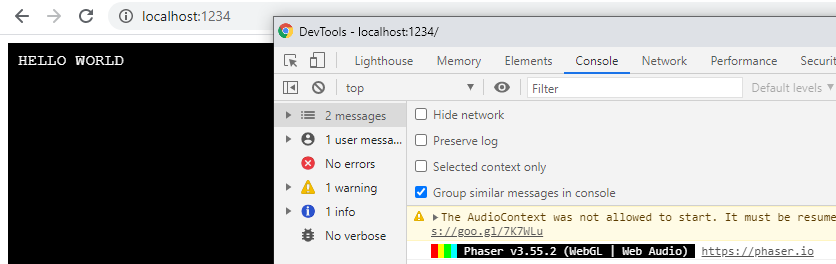
Finally we are able to code a Phaser project with TypeScript, but there is still something to see about the IDE to use and the way to define types, see you next time with the fourth and last part.
Never miss an update! Subscribe, and I will bother you by email only when a new game or full source code comes out.