Talking about HTML5, Javascript, Phaser and TypeScript.
Welcome to the final step of the series about the migration from JavaScript to TypeScript in HTML5 game development using Phaser.
A small recap:
In step 1 we installed and set up Node.js.
In step 2 we covered npm and Parcel.
In step 3 we wrote and ran our first TypeScript Phaser project.
Now it’s time to put all together, and choose an IDE which supports TypeScript and makes our tasks easier.
Visual Studio Code is a free editor available for Windows, macOS and Linux, let’s install it:
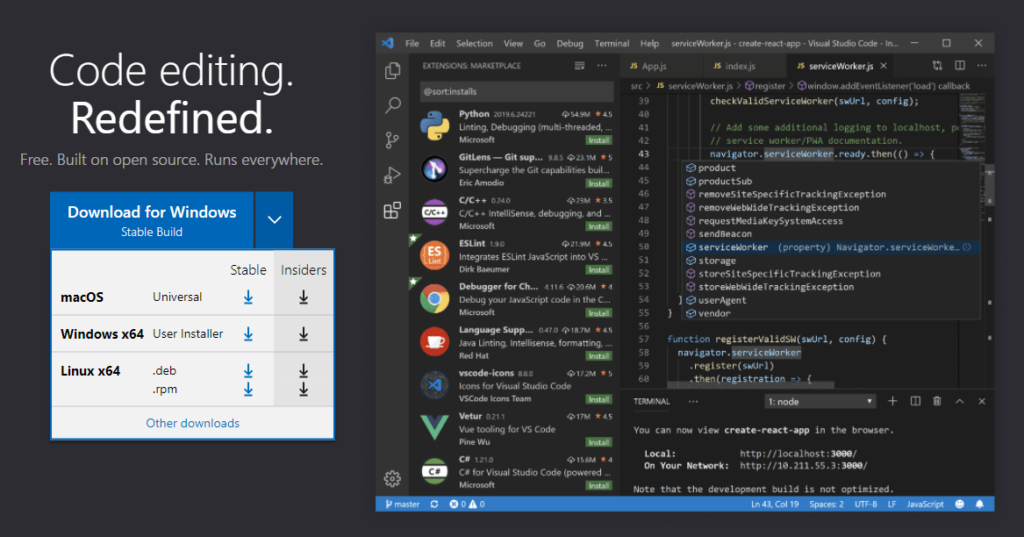
This Visual Studio Code as soon as you open it. Let’s select “Open Folder…”
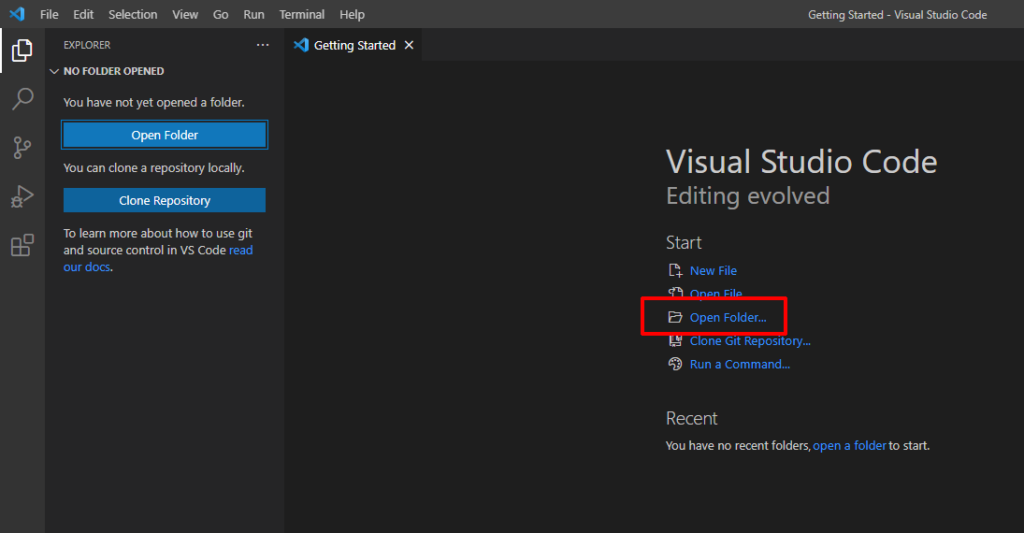
I created an empty folder in my desktop called phaserts
, and selected it.
Now, from “View” menu, open “Terminal” to have your shell directly opened in Visual Studio Code.
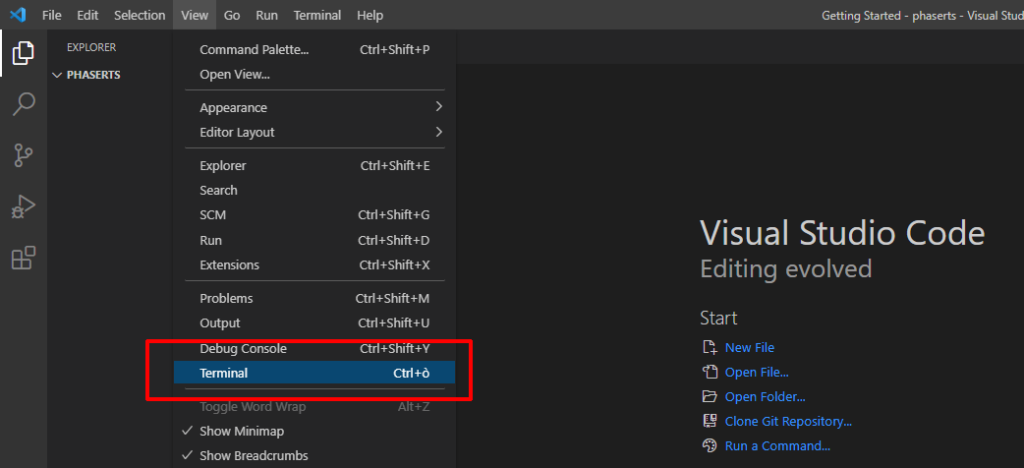
This is how Visual Studio Code should look now:
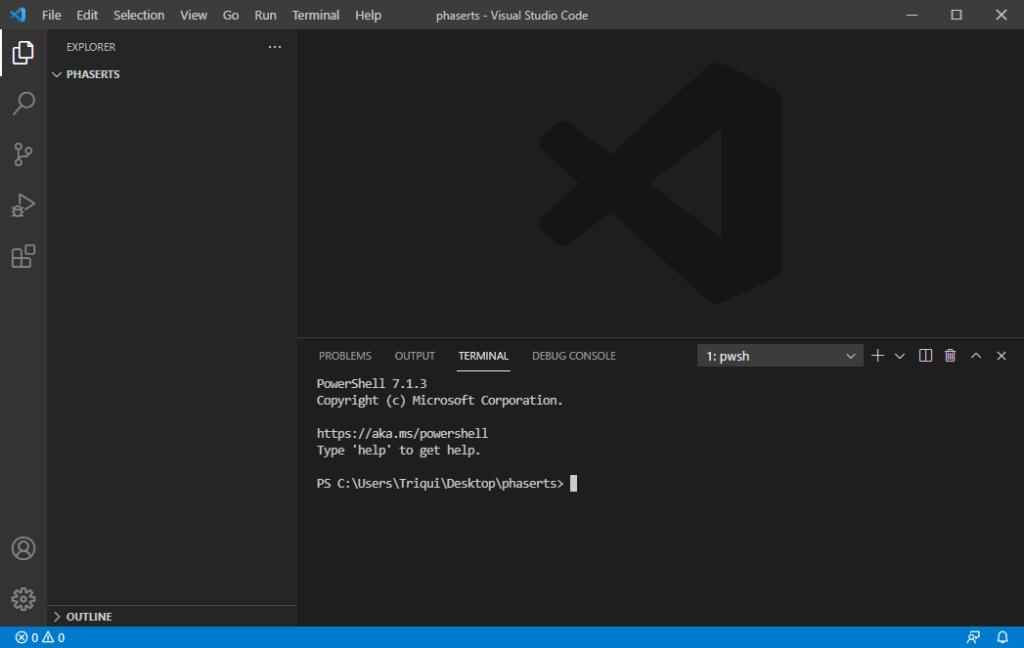
We are now ready to start our project. Just like in step 3, execute in your shell:
npm init -y
then
npm install phaser --save
And this is what you should get:
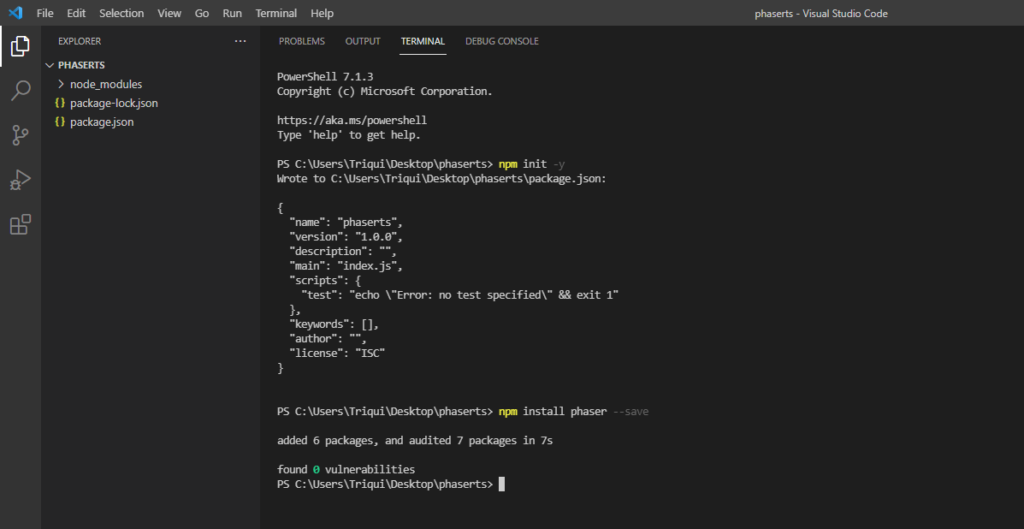
Now, left mouse button in “Explorer” panel and let’s create a new folder:
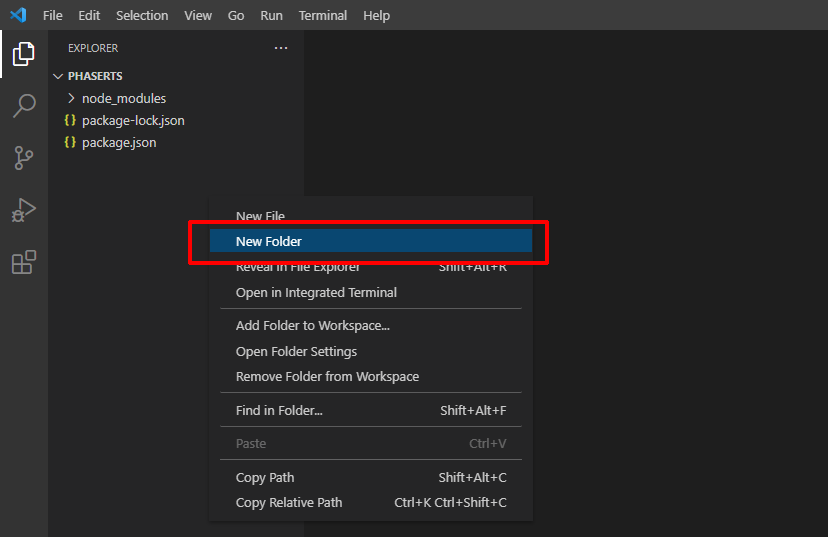
I called the new folder game
, and added the file index.html
, with this content:
<html>
<head>
<title>Hello World</title>
</head>
<body>
<div id = "main"></div>
<script src = "scripts/main.ts"></script>
</body>
</html>
Then, created a subfolder called scripts
, and added main.ts
:
import Phaser from 'phaser'
import { GameScene } from "./gameScene";
const config: Phaser.Types.Core.GameConfig = {
type: Phaser.AUTO,
width: 640,
height: 320,
scene: GameScene
};
new Phaser.Game(config);
It’s important to see how Visual Studio Code helps us both with code completion:

And with inline help:
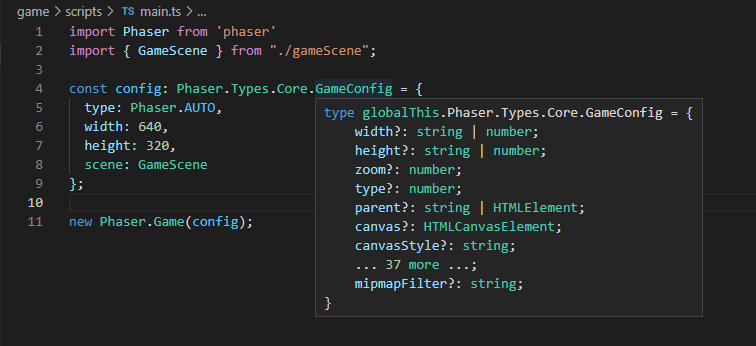
This is thanks to Phaser TypeScript definitions which are automatically loaded in the project. This will make you save a lot of time you would spend browsing the various API docs.
Let’s create gameScene.ts
:
export class GameScene extends Phaser.Scene {
constructor() {
super({
key: "GameScene"
});
}
create() {
this.add.text(10, 10, "HELLO WORLD FROM VISUAL STUDIO CODE");
}
}
Now let’s edit package.json and add a couple of lines:
{
"name": "phaserts",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"parceltest": "parcel ./game/index.html",
"parcelbuild": "parcel build ./game/index.html --out-dir build --no-source-maps --public-url ./"
},
"keywords": [],
"author": "",
"license": "ISC",
"dependencies": {
"phaser": "^3.55.2"
}
}
Line 8 is the “parceltest” script we already encountered in step 3 which tests the game on a local server, while “parcelbuild” at line 9 means “build a distributable version of game/index.html
in build
output directory, don’t create map files and set the public url to current path”.
Now we can write in the shell
npm run parceltest
to run “parcetest” script and test the project, in my case pointing the browser at http://localhost:1234
:
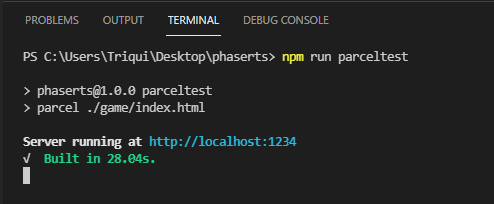
To create a distributable package, you’ll use
npm run parcelbuild
And you will find the files in build
folder:
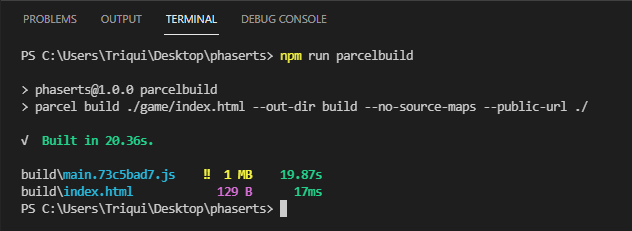
You can then distribute the content of build
folder:

And this is the result:
Now, you have to know Parcel does not support TypeScript’s type checking. This is not a big issue, as most of TypeScript features are supported at IDE level, but just in case you want to be sure you wrote a good and clean TypeScript code, you can init TypeScript by writing in your terminal:
tsc --init
A huge tsconfig.json
file will be created, but it’s not important.
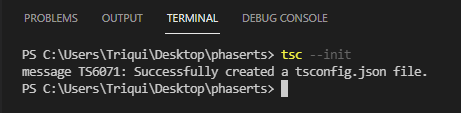
From now on, by writing on the terminal:
tsc --noEmit
You will get absolutely nothing if your TypeScript files are ok, or a list of errors like in this case where I added a “x” to a variable type:
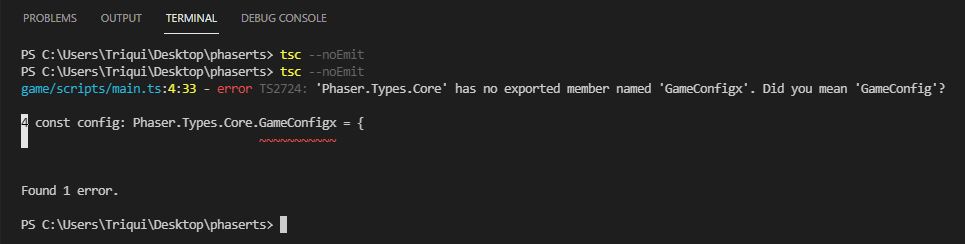
You can automate the process by changing “parcelbuild” script in package.json
:
{
"name": "phaserts",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"parceltest": "parcel ./game/index.html",
"parcelbuild": "tsc --noEmit && parcel build ./game/index.html --out-dir build --no-source-maps --public-url ./"
},
"keywords": [],
"author": "",
"license": "ISC",
"dependencies": {
"phaser": "^3.55.2"
}
}
And now the mingration from JavaScript to TypeScript is over.
Finally, it’s time to start building games again, from now on in TypeScript!!
Never miss an update! Subscribe, and I will bother you by email only when a new game or full source code comes out.