Creation of an HTML5 game like Rise Above using Phaser and Arcade Physics – step 1
Talking about Rise Above game, Game development, HTML5, Javascript and Phaser.
Learn cross platform HTML5 game development
Check my Gumroad page for commented source code, games and books.
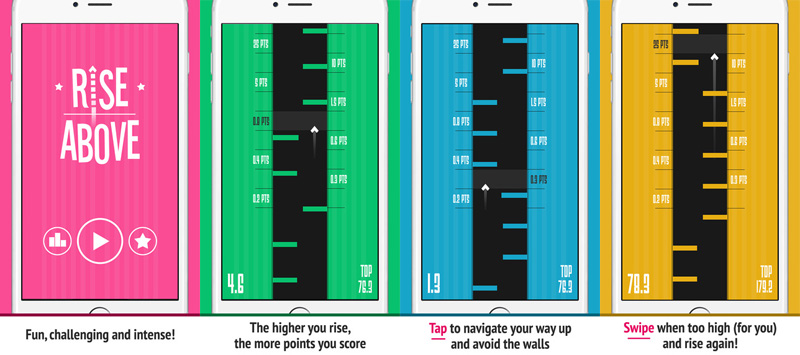
var game;
var ship;
var shipPosition;
var shipPositions;
var shipCanMove;
var shipHorizontalSpeed = 400;
var shipMoveDelay = 500;
window.onload = function() {
game = new Phaser.Game(320, 480, Phaser.AUTO, "");
game.state.add("PlayGame",playGame);
game.state.start("PlayGame");
}
var playGame = function(game){};
playGame.prototype = {
preload: function(){
game.load.image("ship", "ship.png");
},
create: function(){
shipCanMove = true;
shipPosition = 0;
shipPositions = [40, game.width - 40];
game.physics.startSystem(Phaser.Physics.ARCADE);
ship = game.add.sprite(shipPositions[shipPosition], game.height - 40, "ship");
ship.anchor.set(0.5);
game.physics.enable(ship, Phaser.Physics.ARCADE);
ship.body.allowRotation = false;
ship.body.moves = false;
game.input.onDown.add(moveShip);
}
}
function moveShip(){
if(shipCanMove){
shipPosition = 1 - shipPosition;
shipCanMove = false;
var moveTween = game.add.tween(ship).to({
x: shipPositions[shipPosition],
}, shipHorizontalSpeed, Phaser.Easing.Linear.None, true);
moveTween.onComplete.add(function(){
game.time.events.add(shipMoveDelay, function(){
shipCanMove = true;
});
})
}
}
shipHorizontalSpeed
, shipMoveDelay
and shipPositions
to change the gameplay.
Now it’s time to add barriers.
Adding moving barriers and checking collisions
Barriers are created extending sprite class as seen in this post, and we only have to check for collisions between the ship and the group of barriers.
Click on the canvas to move the space ship from one side to another, try to avoid the barriers or the game will restart.
Here is the source code, with new lines highlighted:
var game;
var ship;
var shipPosition;
var shipPositions;
var shipCanMove;
var barrierGroup;
var shipHorizontalSpeed = 400;
var shipMoveDelay = 100;
var barrierSpeed = 120;
var barrierDelay = 2000;
window.onload = function() {
game = new Phaser.Game(320, 480, Phaser.AUTO, "");
game.state.add("PlayGame",playGame);
game.state.start("PlayGame");
}
var playGame = function(game){};
playGame.prototype = {
preload: function(){
game.load.image("ship", "ship.png");
game.load.image("barrier", "barrier.png");
},
create: function(){
shipCanMove = true;
shipPosition = 0;
barrierGroup = game.add.group();
shipPositions = [40, game.width - 40];
game.physics.startSystem(Phaser.Physics.ARCADE);
ship = game.add.sprite(shipPositions[shipPosition], game.height - 40, "ship");
ship.anchor.set(0.5);
game.physics.enable(ship, Phaser.Physics.ARCADE);
ship.body.allowRotation = false;
ship.body.moves = false;
game.input.onDown.add(moveShip);
game.time.events.loop(barrierDelay, function(){
var barrier = new Barrier(game);
game.add.existing(barrier);
barrierGroup.add(barrier);
console.log(barrierGroup);
});
},
update: function(){
game.physics.arcade.collide(ship, barrierGroup, function(){
game.state.start("PlayGame");
});
}
}
function moveShip(){
if(shipCanMove){
shipPosition = 1 - shipPosition;
shipCanMove = false;
var moveTween = game.add.tween(ship).to({
x: shipPositions[shipPosition],
}, shipHorizontalSpeed, Phaser.Easing.Linear.None, true);
moveTween.onComplete.add(function(){
game.time.events.add(shipMoveDelay, function(){
shipCanMove = true;
});
})
}
}
Barrier = function (game) {
var position = game.rnd.between(0, 1);
Phaser.Sprite.call(this, game, game.width * position, -20, "barrier");
game.physics.enable(this, Phaser.Physics.ARCADE);
this.anchor.set(0.5);
};
Barrier.prototype = Object.create(Phaser.Sprite.prototype);
Barrier.prototype.constructor = Barrier;
Barrier.prototype.update = function() {
this.body.velocity.y = barrierSpeed;
if(this.y > game.height){
this.destroy();
}
};
Never miss an update! Subscribe, and I will bother you by email only when a new game or full source code comes out.