Using Tiled editor to create levels and import them as json data in your HTML5 swipe controlled Sokoban game made with Phaser
Talking about Sokoban game, Game development, HTML5, Javascript and Phaser.
Do you like my tutorials?
Then consider supporting me on Ko-fi.
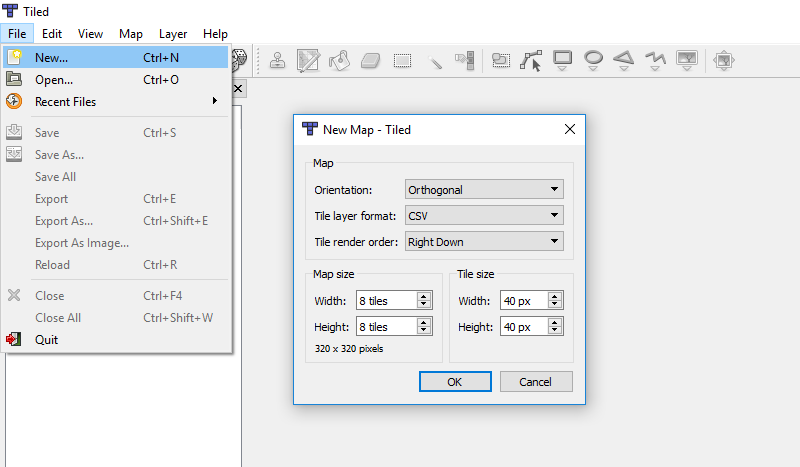
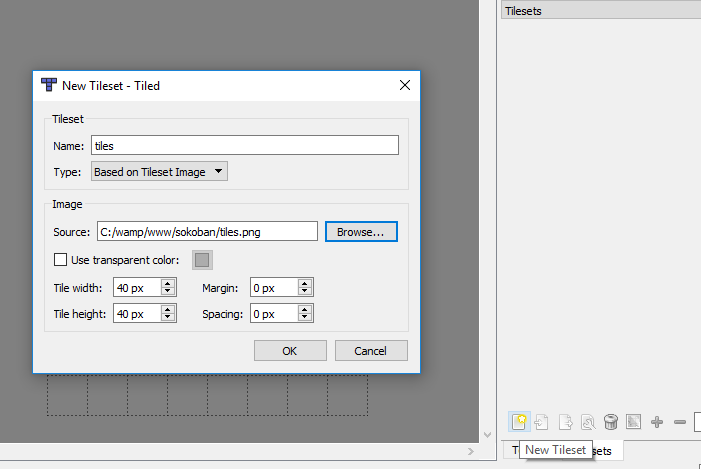
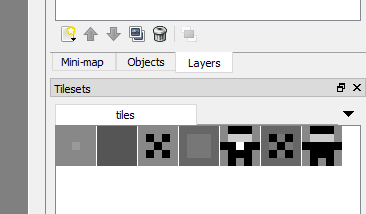
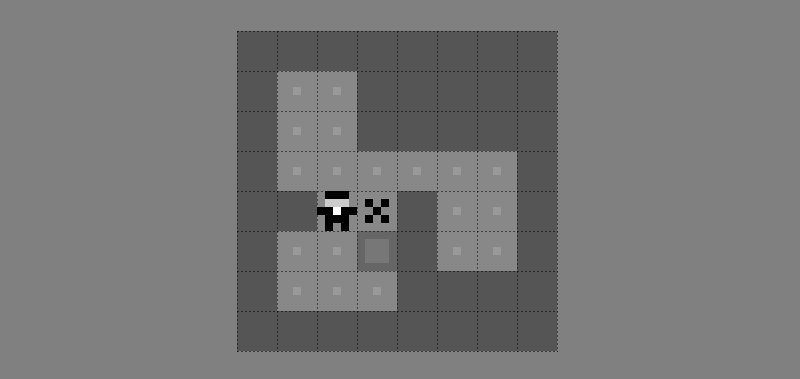
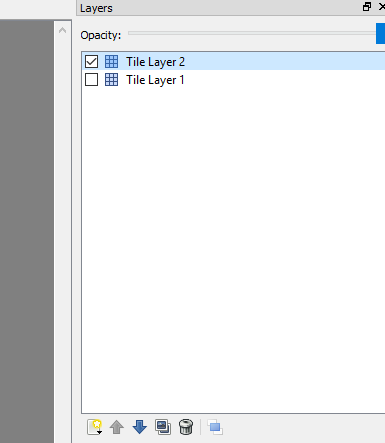
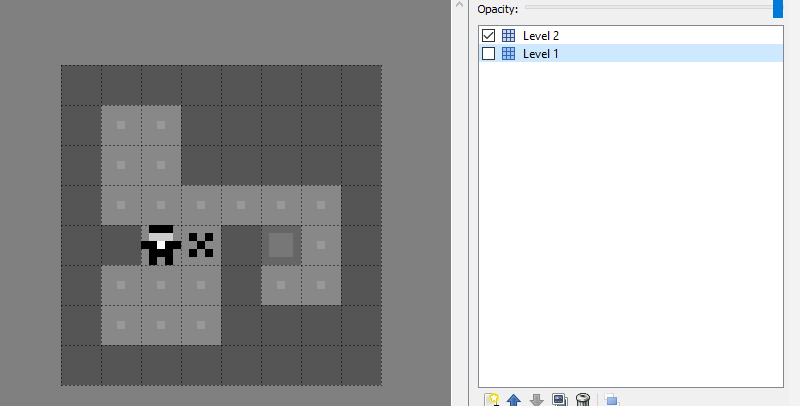
map.json
and put it directly in game folder.
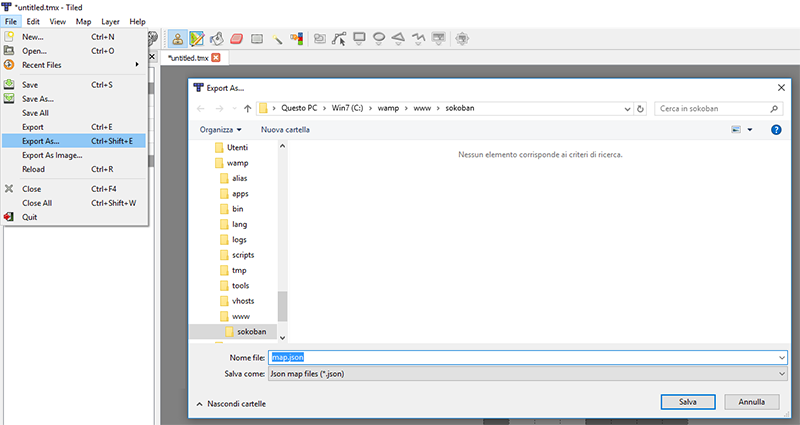
{ "height":8,
"layers":[
{
"data":[2, 2, 2, 2, 2, 2, 2, 2, 2, 1, 1, 2, 2, 2, 2, 2, 2, 1, 1, 2, 2, 2, 2, 2, 2, 1, 1, 1, 1, 1, 1, 2, 2, 2, 5, 3, 2, 1, 1, 2, 2, 1, 1, 4, 2, 1, 1, 2, 2, 1, 1, 1, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
"height":8,
"name":"Level 1",
"opacity":1,
"type":"tilelayer",
"visible":false,
"width":8,
"x":0,
"y":0
},
{
"data":[2, 2, 2, 2, 2, 2, 2, 2, 2, 1, 1, 2, 2, 2, 2, 2, 2, 1, 1, 2, 2, 2, 2, 2, 2, 1, 1, 1, 1, 1, 1, 2, 2, 2, 5, 3, 2, 4, 1, 2, 2, 1, 1, 1, 2, 1, 1, 2, 2, 1, 1, 1, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2],
"height":8,
"name":"Level 2",
"opacity":1,
"type":"tilelayer",
"visible":true,
"width":8,
"x":0,
"y":0
}],
"nextobjectid":1,
"orientation":"orthogonal",
"properties":
{
},
"renderorder":"right-down",
"tileheight":40,
"tilesets":[
{
"firstgid":1,
"image":"tiles.png",
"imageheight":40,
"imagewidth":280,
"margin":0,
"name":"tiles",
"properties":
{
},
"spacing":0,
"tilecount":7,
"tileheight":40,
"tilewidth":40
}],
"tilewidth":40,
"version":1,
"width":8
}
var game;
var gameOptions = {
tileSize: 40,
gameWidth: 320,
gameHeight: 320,
gameSpeed: 100
}
var level = [];
var EMPTY = 0;
var WALL = 1;
var SPOT = 2;
var CRATE = 3;
var PLAYER = 4;
window.onload = function() {
game = new Phaser.Game(gameOptions.gameWidth, gameOptions.gameHeight);
game.state.add("PreloadGame", preloadGame);
game.state.add("PlayGame", playGame);
game.state.start("PreloadGame");
}
var preloadGame = function(game){}
preloadGame.prototype = {
preload: function(){
game.load.spritesheet("tiles", "tiles.png", 40, 40);
game.scale.scaleMode = Phaser.ScaleManager.SHOW_ALL;
game.scale.pageAlignHorizontally = true;
game.scale.pageAlignVertically = true;
game.stage.disableVisibilityChange = true;
var request = new XMLHttpRequest();
request.overrideMimeType("application/json");
request.open("GET", "map.json", true);
request.onreadystatechange = function () {
if (request.readyState == 4 && request.status == "200") {
var jsonLevels = JSON.parse(request.responseText);
for(var k = 0; k < jsonLevels.layers.length; k++){
level[k] = [];
for(var i = 0; i < jsonLevels.layers[k].height; i++){
level[k][i] = [];
for(var j = 0; j < jsonLevels.layers[k].width; j++){
level[k][i][j] = jsonLevels.layers[k].data[i * jsonLevels.layers[k].width + j] - 1;
}
}
}
game.state.start("PlayGame", true, false, 0);
}
};
request.send();
}
}
var playGame = function(game){}
playGame.prototype = {
init: function(currentLevel){
this.currentLevel = currentLevel;
},
create: function(){
this.undoArray = [];
this.crates = [];
this.drawLevel();
game.input.onTap.add(this.handleTap, this);
game.input.onDown.add(this.beginSwipe, this);
},
drawLevel: function(){
this.staticAssetsGroup = game.add.group();
this.movingAssetsGroup = game.add.group();
this.crates.length = 0;
for(var i = 0; i < level[this.currentLevel].length; i++){
this.crates[i] = [];
for(var j = 0; j < level[this.currentLevel][i].length; j++){
this.crates[i][j] = null;
switch(level[this.currentLevel][i][j]){
case PLAYER:
case PLAYER + SPOT:
this.player = game.add.sprite(gameOptions.tileSize * j, gameOptions.tileSize * i, "tiles");
this.player.frame = level[this.currentLevel][i][j];
this.player.posX = j;
this.player.posY = i;
this.movingAssetsGroup.add(this.player);
var tile = game.add.sprite(gameOptions.tileSize * j, gameOptions.tileSize * i, "tiles");
tile.frame = level[this.currentLevel][i][j] - PLAYER;
this.staticAssetsGroup.add(tile);
break;
case CRATE:
case CRATE + SPOT:
this.crates[i][j] = game.add.sprite(gameOptions.tileSize * j, gameOptions.tileSize * i, "tiles");
this.crates[i][j].frame = level[this.currentLevel][i][j];
this.movingAssetsGroup.add(this.crates[i][j]);
var tile = game.add.sprite(gameOptions.tileSize * j, gameOptions.tileSize * i, "tiles");
tile.frame = level[this.currentLevel][i][j] - CRATE;
this.staticAssetsGroup.add(tile);
break;
default:
var tile = game.add.sprite(gameOptions.tileSize * j, gameOptions.tileSize * i, "tiles");
tile.frame = level[this.currentLevel][i][j];
this.staticAssetsGroup.add(tile);
}
}
}
},
handleTap: function(pointer, doubleTap){
if(doubleTap){
if(this.undoArray.length>0){
var undoLevel = this.undoArray.pop();
this.staticAssetsGroup.destroy();
this.movingAssetsGroup.destroy();
level[this.currentLevel] = [];
level[this.currentLevel] = this.copyArray(undoLevel);
this.drawLevel();
}
}
},
beginSwipe: function(e) {
game.input.onDown.remove(this.beginSwipe, this);
game.input.onUp.add(this.endSwipe, this);
},
endSwipe: function(e) {
game.input.onUp.remove(this.endSwipe, this);
var swipeTime = e.timeUp - e.timeDown;
var swipeDistance = Phaser.Point.subtract(e.position, e.positionDown);
var swipeMagnitude = swipeDistance.getMagnitude();
var swipeNormal = Phaser.Point.normalize(swipeDistance);
if(swipeMagnitude > 20 && swipeTime < 1000 && (Math.abs(swipeNormal.y) > 0.8 || Math.abs(swipeNormal.x) > 0.8)) {
if(swipeNormal.x > 0.8) {
this.checkMove(1, 0);
}
if(swipeNormal.x < -0.8) {
this.checkMove(-1, 0);
}
if(swipeNormal.y > 0.8) {
this.checkMove(0, 1);
}
if(swipeNormal.y < -0.8) {
this.checkMove(0, -1);
}
} else {
game.input.onDown.add(this.beginSwipe, this);
}
},
checkMove: function(deltaX, deltaY){
if(this.isWalkable(this.player.posX + deltaX, this.player.posY + deltaY)){
this.undoArray.push(this.copyArray(level[this.currentLevel]));
this.movePlayer(deltaX, deltaY);
return;
}
if(this.isCrate(this.player.posX + deltaX, this.player.posY + deltaY)){
if(this.isWalkable(this.player.posX + 2 * deltaX, this.player.posY + 2 * deltaY)){
this.undoArray.push(this.copyArray(level[this.currentLevel]));
this.moveCrate(deltaX, deltaY);
this.movePlayer(deltaX, deltaY);
return;
}
}
game.input.onDown.add(this.beginSwipe, this);
},
isWalkable: function(posX, posY){
return level[this.currentLevel][posY][posX] == EMPTY || level[this.currentLevel][posY][posX] == SPOT;
},
isCrate: function(posX, posY){
return level[this.currentLevel][posY][posX] == CRATE || level[this.currentLevel][posY][posX] == CRATE + SPOT;
},
movePlayer: function(deltaX, deltaY){
var playerTween =game.add.tween(this.player);
playerTween.to({
x: this.player.x + deltaX * gameOptions.tileSize,
y: this.player.y + deltaY * gameOptions.tileSize
}, gameOptions.gameSpeed, Phaser.Easing.Linear.None, true);
playerTween.onComplete.add(function(){
game.input.onDown.add(this.beginSwipe, this);
this.player.frame = level[this.currentLevel][this.player.posY][this.player.posX];
if(this.isLevelSolved()){
this.currentLevel++;
this.game.state.start("PlayGame", true, false, this.currentLevel);
}
}, this);
level[this.currentLevel][this.player.posY][this.player.posX] -= PLAYER;
this.player.posX += deltaX;
this.player.posY += deltaY;
level[this.currentLevel][this.player.posY][this.player.posX] += PLAYER;
},
moveCrate: function(deltaX, deltaY){
var crateTween = game.add.tween(this.crates[this.player.posY + deltaY][this.player.posX + deltaX]);
crateTween.to({
x: this.crates[this.player.posY + deltaY][this.player.posX + deltaX].x + deltaX * gameOptions.tileSize,
y: this.crates[this.player.posY + deltaY][this.player.posX + deltaX].y + deltaY * gameOptions.tileSize,
}, gameOptions.gameSpeed, Phaser.Easing.Linear.None, true);
crateTween.onComplete.add(function(){
this.crates[this.player.posY + deltaY][this.player.posX + deltaX].frame = level[this.currentLevel][this.player.posY + deltaY][this.player.posX + deltaX];
}, this);
this.crates[this.player.posY + 2 * deltaY][this.player.posX + 2 * deltaX] = this.crates[this.player.posY + deltaY][this.player.posX + deltaX];
this.crates[this.player.posY + deltaY][this.player.posX + deltaX] = null;
level[this.currentLevel][this.player.posY + deltaY][this.player.posX + deltaX] -= CRATE;
level[this.currentLevel][this.player.posY + 2 * deltaY][this.player.posX + 2 * deltaX] += CRATE;
},
isLevelSolved: function(){
for(var i = 0; i < level[this.currentLevel].length; i++){
for(var j = 0; j < level[this.currentLevel][i].length; j++){
if(level[this.currentLevel][i][j] == CRATE){
return false;
}
}
}
return true;
},
copyArray: function(a){
var newArray = a.slice(0);
for(var i = newArray.length; i > 0; i--){
if(newArray[i] instanceof Array){
newArray[i] = this.copyArray(newArray[i]);
}
}
return newArray;
}
}
Never miss an update! Subscribe, and I will bother you by email only when a new game or full source code comes out.